Visual Studio Code (VS Code) is a powerful tool used by developers worldwide. However, you might have noticed variables being grayed out while working on your projects. This can seem confusing, especially if you’re new to programming or using VS Code.
This article will explain why variables appear grayed out in Visual Studio Code and what you can do about it. Whether you’re working with Python, Java, or other languages, understanding this feature can help streamline your coding process.
What Does a Grayed-Out Variable in Visual Studio Code Mean?
A grayed-out variable in VS Code usually indicates that the variable is unused or inactive in your current code. It’s not an error or bug; it’s a feature designed to improve code readability and maintainability.
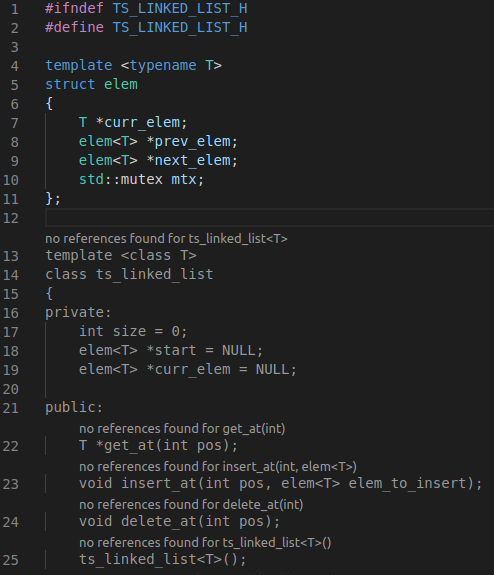
Graying out unused variables helps developers identify unnecessary or redundant code. For instance, if you declare a variable in Python but don’t use it in your program, VS Code will gray it out to signal that it can be removed or modified. Similarly, this applies to other programming languages like Java and JavaScript.
Why Is Variable Grayed Out in Visual Studio Code Python?
In Python, variables are often grayed out because they are declared but never used. For example:
x = 10 # Variable 'x' is declared but never used
In this case, VS Code highlights that x
is unused by graying it out. This behavior can happen due to several reasons:
- Unused Imports or Variables: You might have imported a library or declared variables but forgotten to use them in your code.
- Debugging Leftovers: Sometimes, during debugging, you comment out lines of code that reference a variable. The variable then becomes unused.
- Scopes: Variables declared in a specific scope but not referenced will appear grayed out.
Why Is Variable Grayed Out in Visual Studio Code Example?
To understand this better, let’s look at an example in Python:
import math # 'math' is imported but never used
number = 42 # 'number' is declared but not used
print("Hello, World!")
In this case:
- The
math
library is grayed out because it’s imported but unused. - The variable
number
is also grayed out because it is declared but not utilized.
Such unused variables and imports can clutter your code, making it harder to read. Removing or refactoring them improves the overall quality of your code.
Why Is Variable Grayed Out in Visual Studio Code GitHub?
If you’re working on a project hosted on GitHub and notice grayed-out variables in VS Code, it’s often due to collaborative coding practices. For example:
- Another contributor might have declared a variable or imported a module for a planned feature that wasn’t implemented.
- The code may include placeholders for future updates.
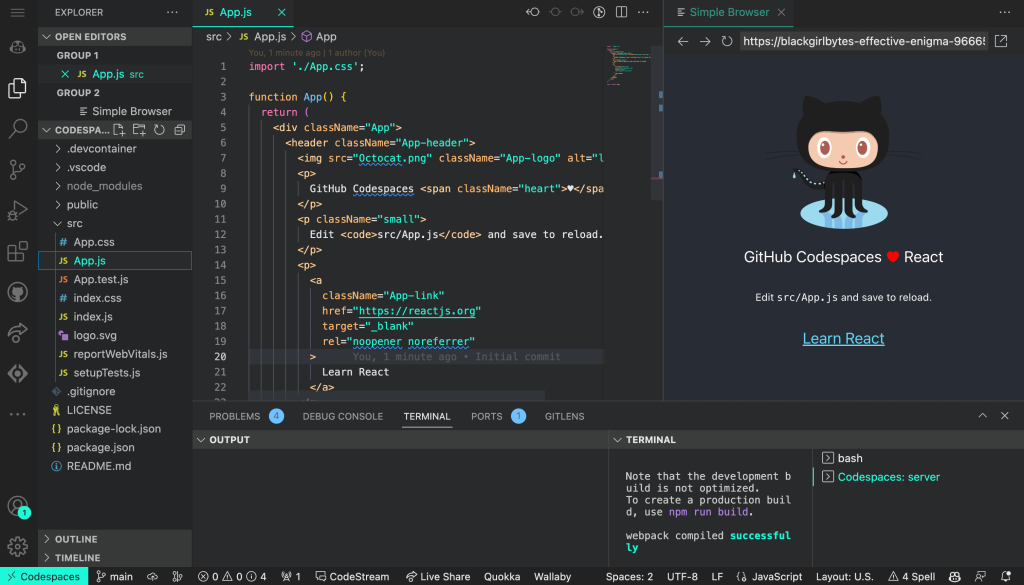
Using GitHub workflows, such as pull requests and code reviews, you can identify and address these grayed-out variables to maintain clean and functional code.
Why Is Variable Grayed Out in Visual Studio Code Java?
In Java, grayed-out variables can occur for similar reasons as in Python:
- Unused Variables: Declared variables that are never referenced.
- Unused Imports: Imported libraries or classes that are not utilized in the code.
Here’s an example in Java:
import java.util.Scanner; // 'Scanner' is imported but not used
public class Main {
public static void main(String[] args) {
int x = 5; // 'x' is declared but not used
System.out.println("Hello, World!");
}
}
The Scanner
class and the variable x
are grayed out in this case, signaling that they serve no purpose in the program.
How to Fix Grayed-Out Variables in VS Code
If you want to resolve or eliminate grayed-out variables in your code, here are some steps to follow:
1. Remove Unused Variables
If a variable is grayed out because it’s not used, consider removing it unless it’s needed for future development.
2. Use the Variable
If the variable is essential, make sure it’s referenced somewhere in your code. For example:
number = 42
print(number) # 'number' is no longer grayed out
3. Disable Linter Rules (If Necessary)
Sometimes, linters like Pylint
or ESLint
flag variables as unused even if you intend to use them later. You can disable these warnings in your settings or code comments:
# pylint: disable=unused-variable
x = 10
4. Configure VS Code Settings
You can tweak VS Code settings to manage how it highlights unused variables. Navigate to:
File > Preferences > Settings > Search: 'Editor Unused Code'
Adjust the settings to suit your preferences.
Vscode Grey Out Unused Variables
Unused variables are commonly highlighted to improve code quality. This feature helps:
- Clean Up Code: Removes unnecessary lines, making the program easier to read.
- Debugging: Quickly identifies placeholders or variables left behind during debugging.
- Best Practices: Encourages writing efficient and maintainable code.
Common Scenarios Where Variables Are Grayed Out
Debugging
Variables might be grayed out after debugging when references to them are commented out or deleted.
Placeholder Variables
Sometimes, developers declare variables for future use but forget to remove them if plans change.
Template Code
In template files or code snippets, some variables may remain unused, leading to grayed-out highlights.
Why Does This Happen Across Different Languages?
The graying-out feature is not limited to Python or Java. It applies to multiple languages like JavaScript, C++, and TypeScript. The primary purpose is to help developers identify unused code regardless of the language.
For example, in JavaScript:
let unusedVariable = 10; // This will be grayed out
console.log("Hello, World!");
Conclusion
Understanding why variables are grayed out in Visual Studio Code helps you write cleaner, more efficient code. Whether you’re coding in Python, Java, or another language, addressing unused variables improves readability and maintainability. Remember that this feature isn’t an error but a helpful tool for developers.
If you’ve ever wondered, “Why is variable grayed out in Visual Studio Code Python?” or looked for solutions like examples and GitHub references, this article provides a clear explanation. Always aim to review and optimize your code to avoid unnecessary clutter and enhance your development process.